Create a Burger Ordering Dashboard with HTML, CSS, and JavaScript
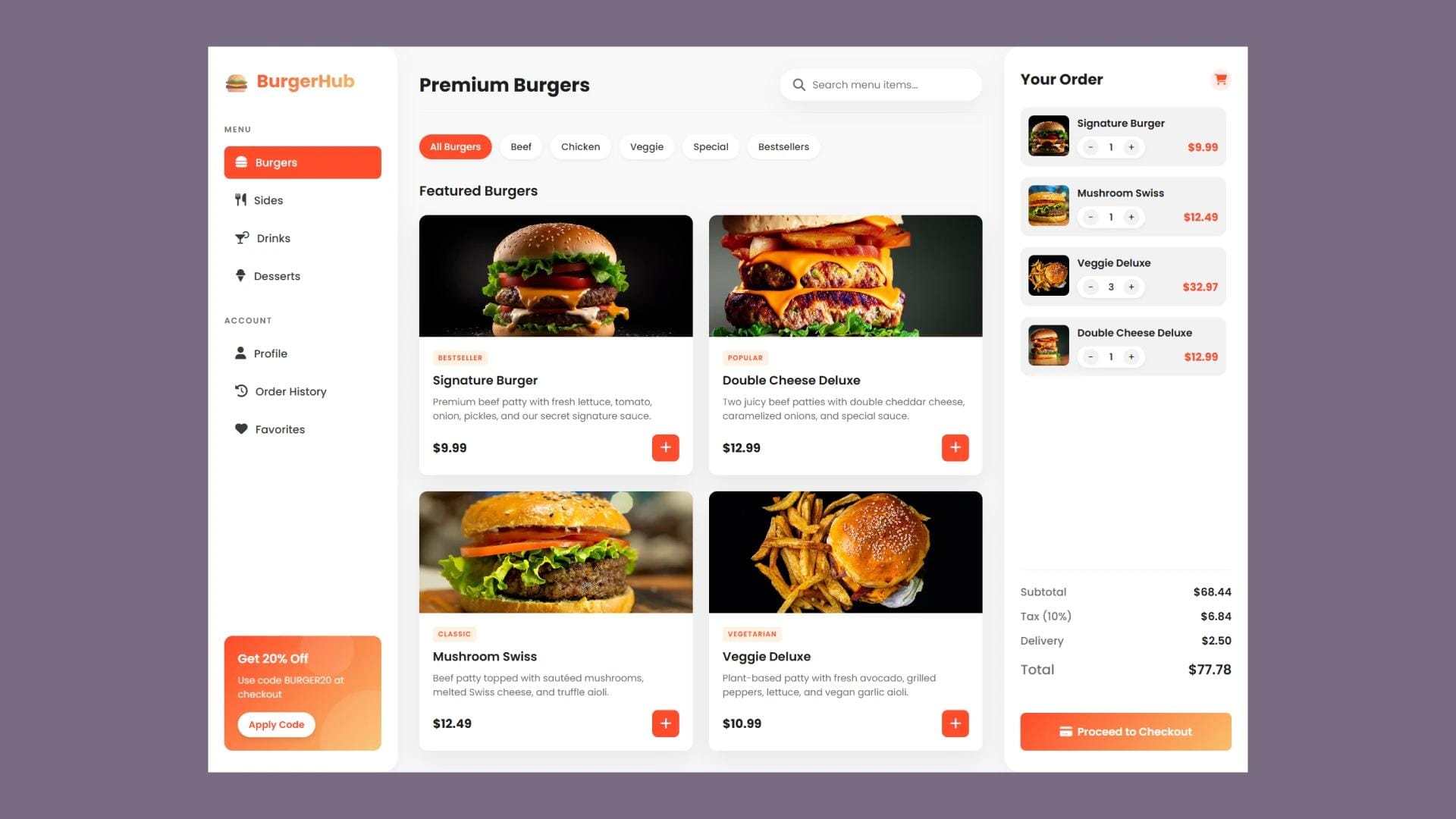
Want to build a simple and interactive burger ordering system? This tutorial will guide you through creating a burger ordering dashboard using HTML, CSS, and JavaScript. Whether you're a beginner or an aspiring web developer, this project will help you learn essential frontend skills while working on a real-world application.
A burger ordering dashboard allows users to browse different burgers, filter them by category, and add their favorite items to the cart. This type of interface is perfect for food-related businesses looking to improve their online ordering experience. Let's dive into how you can build one from scratch!
Features of the Burger Ordering Dashboard
Before we start, let’s look at the key features this dashboard will include:
- User-friendly interface: A visually appealing design with burger images and descriptions.
- Search functionality: Users can quickly find their favorite burgers.
- Filtering options: Sort burgers based on categories like beef, chicken, or vegetarian.
- Interactive shopping cart: Users can add or remove items before placing an order.
- Smooth animations: A modern UI experience with animations for better engagement.
Step-by-Step Guide to Creating the Dashboard
1. Designing the Layout with HTML and CSS
The first step is to structure the dashboard using HTML and style it with CSS. The layout should include a navbar, a burger menu section, a search bar, filtering buttons, and a cart panel.
CSS will be used to create a clean and responsive design, ensuring the dashboard looks great on all devices. You can use flexbox and grid for an organized layout.
2. Implementing Search and Filtering with JavaScript
To enhance user experience, JavaScript will handle the search and filtering functions. Users can type keywords in the search bar, and the dashboard will dynamically display matching results. Similarly, filtering buttons will allow users to view only specific categories of burgers.
3. Adding Items to the Shopping Cart
The shopping cart is a crucial part of any food ordering system. JavaScript will be used to add, remove, and update items in the cart. Whenever a user clicks on the “Add to Cart” button, the item will be stored in an array and displayed in the cart panel.
4. Updating the Cart in Real-Time
Users should see real-time updates whenever they modify their orders. JavaScript’s event listeners will help in updating the cart’s total price and item count dynamically.
5. Enhancing the UI with Animations
To make the dashboard more interactive, simple CSS animations and JavaScript effects can be added. For example, a fade-in effect when items are added to the cart or a sliding menu for the cart panel.
Why Build This Project?
Creating a burger ordering dashboard is a fun and practical project that helps improve frontend development skills. Here’s why it’s a great learning experience:
- Strengthens HTML, CSS, and JavaScript skills.
- Enhances UI/UX design abilities.
- Teaches real-world application development.
- Provides a foundation for building advanced e-commerce websites.
Source Code
- Step 1: Setting Up the HTML Structure
- Step 2: Adding Styling with CSS
- Step 3: Adding Interactivity with JavaScript
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8" /><meta name="viewport" content="width=device-width, initial-scale=1.0" /><title>Burger Dashboard</title><link rel="preconnect" href="https://fonts.googleapis.com" /><link rel="preconnect" href="https://fonts.gstatic.com" crossorigin /><linkhref="https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600;700&display=swap"rel="stylesheet"/><linkrel="stylesheet"href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.0/css/all.min.css"/><link rel="stylesheet" href="styles.css" /></head><body><div class="dashboard"><!-- Sidebar / Categories --><div class="sidebar"><div class="logo"><imgsrc="https://api.iconify.design/fluent-emoji:hamburger.svg"class="logo-icon"alt="Logo"/><span>BurgerHub</span></div><div class="nav-section"><h4 class="nav-header">Menu</h4><div class="nav-item active"><i class="fas fa-hamburger"></i><span>Burgers</span></div><div class="nav-item"><i class="fas fa-utensils"></i><span>Sides</span></div><div class="nav-item"><i class="fas fa-cocktail"></i><span>Drinks</span></div><div class="nav-item"><i class="fas fa-ice-cream"></i><span>Desserts</span></div></div><div class="nav-section"><h4 class="nav-header">Account</h4><div class="nav-item"><i class="fas fa-user"></i><span>Profile</span></div><div class="nav-item"><i class="fas fa-history"></i><span>Order History</span></div><div class="nav-item"><i class="fas fa-heart"></i><span>Favorites</span></div></div><div class="promo-card"><h3 class="promo-title">Get 20% Off</h3><p class="promo-description">Use code BURGER20 at checkout</p><button class="promo-button">Apply Code</button></div></div><!-- Main Content Area --><div class="main-content"><div class="header"><h1 class="page-title">Premium Burgers</h1><div class="search-container"><i class="fas fa-search search-icon"></i><inputtype="text"class="search-input"placeholder="Search menu items..."/></div></div><div class="categories-filters"><div class="filter-chip active">All Burgers</div><div class="filter-chip">Beef</div><div class="filter-chip">Chicken</div><div class="filter-chip">Veggie</div><div class="filter-chip">Special</div><div class="filter-chip">Bestsellers</div></div><h2 class="section-title">Featured Burgers</h2><!-- Menu Items --><div class="menu-items"><div class="menu-item" data-id="1"><imgsrc="https://cdn.pixabay.com/photo/2023/09/25/22/08/ai-generated-8276129_1280.jpg"alt="Signature Burger"class="item-image"/><div class="item-details"><div class="item-tags"><span class="item-tag">Bestseller</span></div><h3 class="item-name">Signature Burger</h3><p class="item-description">Premium beef patty with fresh lettuce, tomato, onion, pickles,and our secret signature sauce.</p><div class="item-bottom"><span class="item-price">$9.99</span><button class="add-to-cart"><i class="fas fa-plus"></i></button></div></div></div><div class="menu-item" data-id="2"><imgsrc="https://cdn.pixabay.com/photo/2022/08/29/17/44/burger-7419420_1280.jpg"alt="Double Cheese Deluxe"class="item-image"/><div class="item-details"><div class="item-tags"><span class="item-tag">Popular</span></div><h3 class="item-name">Double Cheese Deluxe</h3><p class="item-description">Two juicy beef patties with double cheddar cheese, caramelizedonions, and special sauce.</p><div class="item-bottom"><span class="item-price">$12.99</span><button class="add-to-cart"><i class="fas fa-plus"></i></button></div></div></div><div class="menu-item" data-id="3"><imgsrc="https://cdn.pixabay.com/photo/2014/10/19/20/59/hamburger-494706_1280.jpg"alt="Bacon Avocado"class="item-image"/><div class="item-details"><div class="item-tags"><span class="item-tag">New</span></div><h3 class="item-name">Bacon Avocado</h3><p class="item-description">Angus beef patty topped with crispy bacon, fresh avocado,lettuce, and garlic aioli.</p><div class="item-bottom"><span class="item-price">$13.99</span><button class="add-to-cart"><i class="fas fa-plus"></i></button></div></div></div><div class="menu-item" data-id="4"><imgsrc="https://cdn.pixabay.com/photo/2020/09/14/16/23/burger-5571385_1280.jpg"alt="Spicy Jalapeño"class="item-image"/><div class="item-details"><div class="item-tags"><span class="item-tag">Spicy</span></div><h3 class="item-name">Spicy Jalapeño</h3><p class="item-description">Beef patty with pepper jack cheese, fresh jalapeños, spicy mayo,and crispy onion strings.</p><div class="item-bottom"><span class="item-price">$11.99</span><button class="add-to-cart"><i class="fas fa-plus"></i></button></div></div></div><div class="menu-item" data-id="5"><imgsrc="https://cdn.pixabay.com/photo/2021/12/09/20/46/burger-6859072_1280.jpg"alt="Mushroom Swiss"class="item-image"/><div class="item-details"><div class="item-tags"><span class="item-tag">Classic</span></div><h3 class="item-name">Mushroom Swiss</h3><p class="item-description">Beef patty topped with sautéed mushrooms, melted Swiss cheese,and truffle aioli.</p><div class="item-bottom"><span class="item-price">$12.49</span><button class="add-to-cart"><i class="fas fa-plus"></i></button></div></div></div><div class="menu-item" data-id="6"><imgsrc="https://cdn.pixabay.com/photo/2021/03/19/21/52/burger-6108495_1280.jpg"alt="Veggie Deluxe"class="item-image"/><div class="item-details"><div class="item-tags"><span class="item-tag">Vegetarian</span></div><h3 class="item-name">Veggie Deluxe</h3><p class="item-description">Plant-based patty with fresh avocado, grilled peppers, lettuce,and vegan garlic aioli.</p><div class="item-bottom"><span class="item-price">$10.99</span><button class="add-to-cart"><i class="fas fa-plus"></i></button></div></div></div></div></div><!-- Cart Section --><div class="cart-section"><div class="cart-title"><span>Your Order</span><div class="cart-icon"><i class="fas fa-shopping-cart"></i></div></div><div class="cart-items"><!-- Empty cart message --><div class="empty-cart"><div class="empty-cart-icon"><i class="fas fa-shopping-bag"></i></div><p>Your cart is empty</p><p>Add some delicious burgers to get started</p></div><!-- Cart items will be added here by JavaScript --></div><div class="cart-total"><div class="total-row"><span class="total-title">Subtotal</span><span class="total-value" id="subtotal">$0.00</span></div><div class="total-row"><span class="total-title">Tax (10%)</span><span class="total-value" id="tax">$0.00</span></div><div class="total-row"><span class="total-title">Delivery</span><span class="total-value" id="delivery">$2.50</span></div><div class="total-row grand-total"><span class="total-title">Total</span><span class="total-value" id="total">$0.00</span></div></div><button class="checkout-btn"><i class="fas fa-credit-card"></i>Proceed to Checkout</button></div></div><script src="script.js"></script></body></html>
:root {--primary: #ff4b2b;--primary-hover: #ff7857;--secondary: #f9c06a;--dark: #1d1d1d;--text: #3a3a3a;--light-text: #717171;--light: #f8f8f8;--white: #ffffff;--off-white: #f2f2f2;--shadow: 0 10px 30px rgba(0, 0, 0, 0.05);--card-shadow: 0 15px 35px rgba(0, 0, 0, 0.07);--hover-shadow: 0 15px 35px rgba(0, 0, 0, 0.1);--border-radius: 16px;--card-radius: 12px;--button-radius: 8px;--transition: all 0.3s cubic-bezier(0.25, 0.8, 0.25, 1);}* {margin: 0;padding: 0;box-sizing: border-box;font-family: "Poppins", sans-serif;}body {background-color: var(--light);color: var(--text);display: flex;}.dashboard {display: flex;width: 100%;max-width: 1600px;margin: 0 auto;}/* Sidebar Styles */.sidebar {width: 280px;background-color: var(--white);box-shadow: var(--shadow);padding: 32px 24px;display: flex;flex-direction: column;z-index: 10;border-radius: 0 24px 24px 0;position: fixed;top: 0;bottom: 0;left: 0;}.logo {font-size: 26px;font-weight: 700;color: var(--dark);margin-bottom: 42px;display: flex;align-items: center;gap: 12px;}.logo span {background: linear-gradient(135deg, var(--primary) 0%, var(--secondary) 100%);-webkit-background-clip: text;-webkit-text-fill-color: transparent;}.logo-icon {width: 36px;height: 36px;}.nav-section {margin-bottom: 24px;}.nav-header {font-size: 12px;text-transform: uppercase;letter-spacing: 1.5px;color: var(--light-text);margin-bottom: 16px;font-weight: 600;}.nav-item {display: flex;align-items: center;padding: 12px 16px;margin-bottom: 8px;border-radius: var(--button-radius);cursor: pointer;transition: var(--transition);font-weight: 500;color: var(--text);}.nav-item:hover {background-color: var(--off-white);}.nav-item.active {background-color: var(--primary);color: var(--white);}.nav-item i {margin-right: 12px;font-size: 18px;}.promo-card {background: linear-gradient(135deg, var(--primary) 0%, var(--secondary) 100%);border-radius: var(--card-radius);padding: 20px;margin-top: auto;color: white;position: relative;overflow: hidden;}.promo-title {font-weight: 600;font-size: 18px;margin-bottom: 8px;}.promo-description {font-size: 14px;margin-bottom: 16px;opacity: 0.9;}.promo-button {background-color: white;color: var(--primary);border: none;padding: 8px 16px;border-radius: 20px;font-weight: 600;font-size: 14px;cursor: pointer;box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);transition: var(--transition);}.promo-button:hover {transform: translateY(-2px);box-shadow: 0 6px 12px rgba(0, 0, 0, 0.15);}.promo-card::after {content: "";position: absolute;width: 150px;height: 150px;background-color: rgba(255, 255, 255, 0.1);border-radius: 50%;right: -60px;bottom: -60px;}.promo-card::before {content: "";position: absolute;width: 80px;height: 80px;background-color: rgba(255, 255, 255, 0.1);border-radius: 50%;right: 40px;top: -20px;}/* Main Content Styles */.main-content {flex: 1;padding: 32px;display: flex;flex-direction: column;background-color: var(--light);margin-left: 280px;margin-right: 360px;}.header {display: flex;justify-content: space-between;align-items: center;margin-bottom: 32px;padding-bottom: 16px;border-bottom: 1px solid rgba(0, 0, 0, 0.05);}.page-title {font-size: 28px;font-weight: 700;color: var(--dark);}.search-container {position: relative;}.search-input {background-color: var(--white);border: 1px solid rgba(0, 0, 0, 0.05);padding: 12px 20px 12px 48px;border-radius: 30px;width: 300px;font-size: 15px;box-shadow: var(--shadow);transition: var(--transition);}.search-input:focus {outline: none;border-color: var(--primary);box-shadow: 0 0 0 3px rgba(255, 75, 43, 0.1);}.search-icon {position: absolute;left: 20px;top: 50%;transform: translateY(-50%);color: var(--light-text);font-size: 18px;}.categories-filters {display: flex;gap: 12px;margin-bottom: 24px;/* overflow-x: auto; */padding-bottom: 8px;}.filter-chip {padding: 8px 16px;background-color: var(--white);border-radius: 20px;font-size: 14px;font-weight: 500;cursor: pointer;transition: var(--transition);white-space: nowrap;box-shadow: 0 2px 5px rgba(0, 0, 0, 0.05);}.filter-chip:hover {background-color: var(--off-white);}.filter-chip.active {background-color: var(--primary);color: white;}.section-title {font-size: 20px;font-weight: 600;margin-bottom: 20px;color: var(--dark);}.menu-items {display: grid;grid-template-columns: repeat(auto-fill, minmax(280px, 1fr));gap: 24px;}.menu-item {background-color: var(--white);border-radius: var(--card-radius);overflow: hidden;box-shadow: var(--card-shadow);transition: var(--transition);display: flex;flex-direction: column;}.menu-item:hover {transform: translateY(-5px);box-shadow: var(--hover-shadow);}.item-image {height: 180px;width: 100%;object-fit: cover;transition: transform 0.5s ease;}.menu-item:hover .item-image {transform: scale(1.05);}.item-details {padding: 20px;flex: 1;display: flex;flex-direction: column;}.item-tags {display: flex;gap: 6px;margin-bottom: 8px;}.item-tag {font-size: 10px;text-transform: uppercase;letter-spacing: 1px;background-color: rgba(249, 192, 106, 0.2);color: var(--primary);padding: 4px 8px;border-radius: 4px;font-weight: 600;}.item-name {font-weight: 600;margin-bottom: 8px;font-size: 18px;color: var(--dark);}.item-description {color: var(--light-text);font-size: 14px;margin-bottom: 16px;line-height: 1.5;flex: 1;}.item-bottom {display: flex;justify-content: space-between;align-items: center;margin-top: auto;}.item-price {font-weight: 700;color: var(--dark);font-size: 18px;}.add-to-cart {background-color: var(--primary);color: white;border: none;border-radius: var(--button-radius);width: 40px;height: 40px;display: flex;align-items: center;justify-content: center;cursor: pointer;transition: var(--transition);font-size: 18px;}.add-to-cart:hover {background-color: var(--primary-hover);transform: scale(1.05);}/* Cart Styles */.cart-section {width: 360px;background-color: var(--white);box-shadow: var(--shadow);padding: 32px 24px;display: flex;flex-direction: column;border-radius: 24px 0 0 24px;position: fixed;top: 0;right: 0;height: 100vh;}.cart-title {font-size: 22px;font-weight: 700;margin-bottom: 24px;color: var(--dark);display: flex;justify-content: space-between;align-items: center;}.cart-icon {width: 32px;height: 32px;background-color: rgba(255, 75, 43, 0.1);color: var(--primary);border-radius: 50%;display: flex;align-items: center;justify-content: center;font-size: 16px;}.cart-items {flex: 1;overflow-y: auto;display: flex;flex-direction: column;gap: 16px;margin-bottom: 24px;padding-right: 8px;}.cart-items::-webkit-scrollbar {width: 4px;}.cart-items::-webkit-scrollbar-thumb {background-color: rgba(0, 0, 0, 0.1);border-radius: 4px;}.cart-item {display: flex;background-color: var(--off-white);border-radius: var(--card-radius);padding: 12px;transition: var(--transition);}.cart-item:hover {background-color: rgba(255, 75, 43, 0.05);}.cart-item-image {width: 60px;height: 60px;border-radius: 8px;margin-right: 12px;object-fit: cover;}.cart-item-details {flex: 1;}.cart-item-name {font-weight: 600;margin-bottom: 4px;font-size: 15px;color: var(--dark);}.cart-item-actions {display: flex;justify-content: space-between;align-items: center;margin-top: 8px;}.quantity-control {display: flex;align-items: center;gap: 8px;background-color: white;border-radius: 20px;padding: 4px 8px;box-shadow: 0 2px 5px rgba(0, 0, 0, 0.05);}.quantity-btn {width: 24px;height: 24px;background-color: var(--off-white);border: none;border-radius: 50%;display: flex;align-items: center;justify-content: center;cursor: pointer;font-size: 14px;transition: var(--transition);}.quantity-btn:hover {background-color: var(--primary);color: white;}.quantity {font-size: 14px;font-weight: 600;width: 20px;text-align: center;}.cart-item-price {font-weight: 700;color: var(--primary);font-size: 16px;position: absolute;right: 1px;bottom: 1px;padding: 4px;}.cart-total {padding: 20px 0;border-top: 1px solid rgba(0, 0, 0, 0.05);}.total-row {display: flex;justify-content: space-between;margin-bottom: 12px;}.total-title {color: var(--light-text);font-weight: 500;}.total-value {font-weight: 600;color: var(--dark);}.grand-total {font-size: 20px;font-weight: 700;color: var(--primary);margin-top: 16px;}.checkout-btn {background: linear-gradient(135deg, var(--primary) 0%, var(--secondary) 100%);color: white;border: none;border-radius: var(--button-radius);padding: 16px;font-weight: 600;font-size: 16px;cursor: pointer;transition: var(--transition);margin-top: 16px;display: flex;align-items: center;justify-content: center;gap: 8px;}.checkout-btn:hover {transform: translateY(-2px);box-shadow: 0 6px 12px rgba(255, 75, 43, 0.2);}.empty-cart {text-align: center;color: var(--light-text);margin: 48px 0;font-size: 14px;}.empty-cart-icon {font-size: 48px;margin-bottom: 16px;opacity: 0.3;}/* Icons using Font Awesome */@import url("https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.4/css/all.min.css");/* Mobile responsiveness */@media (max-width: 1200px) {.cart-section {width: 320px;}.sidebar {width: 240px;}}@media (max-width: 992px) {.dashboard {flex-direction: column;height: auto;}.sidebar {width: 100%;border-radius: 0;padding: 20px;flex-direction: row;justify-content: space-between;align-items: center;flex-wrap: wrap;}.logo {margin-bottom: 0;}.nav-section {display: none;}.promo-card {display: none;}.main-content {padding: 20px;}.cart-section {width: 100%;border-radius: 0;}}@media (max-width: 768px) {.menu-items {grid-template-columns: repeat(auto-fill, minmax(240px, 1fr));gap: 16px;}.header {flex-direction: column;align-items: flex-start;gap: 16px;}.search-container {width: 100%;}.search-input {width: 100%;}}
// Store menu items dataconst menuItems = [{id: 1,name: "Signature Burger",description:"Premium beef patty with fresh lettuce, tomato, onion, pickles, and our secret signature sauce.",price: 9.99,category: "burgers",image:"https://cdn.pixabay.com/photo/2023/09/25/22/08/ai-generated-8276129_1280.jpg",tags: ["Bestseller"],},{id: 2,name: "Double Cheese Deluxe",description:"Two juicy beef patties with double cheddar cheese, caramelized onions, and special sauce.",price: 12.99,category: "burgers",image:"https://cdn.pixabay.com/photo/2022/08/29/17/44/burger-7419420_1280.jpg",tags: ["Popular"],},{id: 3,name: "Bacon Avocado",description:"Angus beef patty topped with crispy bacon, fresh avocado, lettuce, and garlic aioli.",price: 13.99,category: "burgers",image:"https://cdn.pixabay.com/photo/2014/10/19/20/59/hamburger-494706_1280.jpg",tags: ["New"],},{id: 4,name: "Spicy Jalapeño",description:"Beef patty with pepper jack cheese, fresh jalapeños, spicy mayo, and crispy onion strings.",price: 11.99,category: "burgers",image:"https://cdn.pixabay.com/photo/2020/09/14/16/23/burger-5571385_1280.jpg",tags: ["Spicy"],},{id: 5,name: "Mushroom Swiss",description:"Beef patty topped with sautéed mushrooms, melted Swiss cheese, and truffle aioli.",price: 12.49,category: "burgers",image:"https://cdn.pixabay.com/photo/2021/12/09/20/46/burger-6859072_1280.jpg",tags: ["Classic"],},{id: 6,name: "Veggie Deluxe",description:"Plant-based patty with fresh avocado, grilled peppers, lettuce, and vegan garlic aioli.",price: 10.99,category: "burgers",image:"https://cdn.pixabay.com/photo/2021/03/19/21/52/burger-6108495_1280.jpg",tags: ["Vegetarian"],},];// Cart statelet cart = [];// DOM elementsconst cartItemsEl = document.querySelector(".cart-items");const emptyCartEl = document.querySelector(".empty-cart");const searchInput = document.querySelector(".search-input");const filterChips = document.querySelectorAll(".filter-chip");// Initializedocument.addEventListener("DOMContentLoaded", () => {document.querySelectorAll(".add-to-cart").forEach((button) => {button.addEventListener("click", (e) => {const menuItem = e.target.closest(".menu-item");const itemId = parseInt(menuItem.dataset.id);addToCart(itemId);});});searchInput.addEventListener("input", searchItems);filterChips.forEach((chip) => {chip.addEventListener("click", () => {filterChips.forEach((c) => c.classList.remove("active"));chip.classList.add("active");});});});// Search itemsfunction searchItems() {const searchTerm = searchInput.value.toLowerCase().trim();document.querySelectorAll(".menu-item").forEach((item) => {// Get the item detailsconst name = item.querySelector(".item-name").textContent.toLowerCase();const description = item.querySelector(".item-description").textContent.toLowerCase();if (name.includes(searchTerm) || description.includes(searchTerm)) {item.style.display = "block";} else {item.style.display = "none";}});}// Add item to cartfunction addToCart(itemId) {const item = menuItems.find((item) => item.id === itemId);if (item) {const existingItem = cart.find((cartItem) => cartItem.id === itemId);if (existingItem) {existingItem.quantity += 1;} else {cart.push({ ...item, quantity: 1 });}updateCart();}}// Remove item from cartfunction removeFromCart(itemId) {const itemIndex = cart.findIndex((item) => item.id === itemId);if (itemIndex !== -1) {if (cart[itemIndex].quantity > 1) {cart[itemIndex].quantity -= 1;} else {cart.splice(itemIndex, 1);}// Update the cart UIupdateCart();}}// Update cart UIfunction updateCart() {// Show/hide empty cart messageif (cart.length === 0) {emptyCartEl.style.display = "block";} else {emptyCartEl.style.display = "none";}// Clear the cart items container (except the empty cart message)const cartItemsToRemove = cartItemsEl.querySelectorAll(".cart-item");cartItemsToRemove.forEach((item) => item.remove());// Add each cart item to the UIcart.forEach((item) => {const cartItemEl = document.createElement("div");cartItemEl.className = "cart-item";cartItemEl.innerHTML = `<img src="${item.image}" alt="${item.name}" class="cart-item-image"><div class="cart-item-details"><div class="cart-item-name">${item.name}</div><div class="cart-item-actions"><div class="quantity-control"><button class="quantity-btn decrease" data-id="${item.id}">-</button><span class="quantity">${item.quantity}</span><button class="quantity-btn increase" data-id="${item.id}">+</button></div><span class="cart-item-price">$${(item.price * item.quantity).toFixed(2)}</span></div></div>`;// Add to cart items containercartItemsEl.appendChild(cartItemEl);// Add event listeners for quantity buttonscartItemEl.querySelector(".decrease").addEventListener("click", () => {removeFromCart(item.id);});cartItemEl.querySelector(".increase").addEventListener("click", () => {addToCart(item.id);});});// Update totalsupdateTotals();}// Update the cart totalsfunction updateTotals() {// Calculate subtotalconst subtotal = cart.reduce((total, item) => total + item.price * item.quantity,0);const tax = subtotal * 0.1; // 10% taxconst delivery = cart.length > 0 ? 2.5 : 0;const total = subtotal + tax + delivery;// Update the UIdocument.getElementById("subtotal").textContent = `$${subtotal.toFixed(2)}`;document.getElementById("tax").textContent = `$${tax.toFixed(2)}`;document.getElementById("delivery").textContent =cart.length > 0 ? `$2.50` : `$0.00`;document.getElementById("total").textContent = `$${total.toFixed(2)}`;}
Conclusion
A burger ordering dashboard built with HTML, CSS, and JavaScript is a great beginner-friendly project. It includes essential web development concepts like UI design, interactivity, and user experience. By following this guide, you’ll gain hands-on experience that will be valuable for future projects.
So, get started today and build an interactive burger ordering system that looks professional and functions smoothly!
Web Developer and Content Creator