How to Make a Finance Dashboard with HTML and CSS
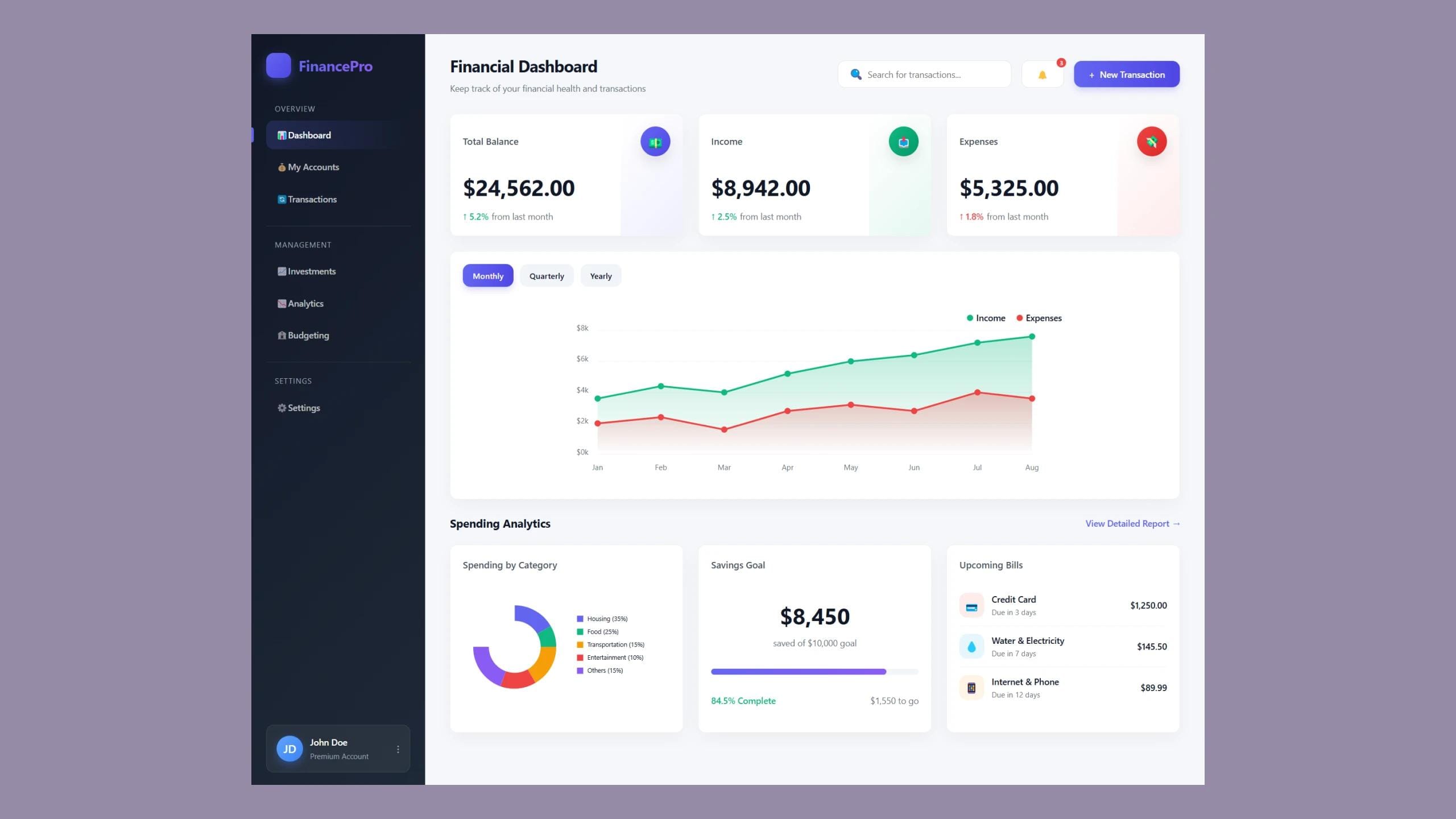
A finance dashboard is an essential tool for tracking income, expenses, savings, and investments in one place. Whether you’re managing personal finances or overseeing business accounts, a well-structured finance dashboard helps you analyze financial data efficiently. In this guide, we’ll explore how to build a responsive finance dashboard using HTML and CSS, ensuring a seamless and user-friendly experience.
Why Build a Finance Dashboard?
A well-designed finance dashboard offers several advantages:
- Financial Insights: Get a clear picture of income, expenses, and savings.
- Better Budgeting: Track spending habits and plan finances effectively.
- User-Friendly Interface: Navigate financial data easily with an intuitive layout.
- Responsive Design: Access your dashboard on any device.
Key Features of a Finance Dashboard
Before you start, consider adding the following features to your finance dashboard:
- Overview Section: A summary of financial status with balance, income, and expenses.
- Transaction History: A detailed list of all recent transactions.
- Budgeting Tools: Categories for tracking expenses and setting budget limits.
- Analytics and Charts: Visual representations of financial data for better understanding.
- Account Management: Separate sections for savings, investments, and loans.
Steps to Build a Finance Dashboard
1. Plan the Structure of Your Dashboard
Define the sections and layout of your finance dashboard before coding. Essential pages include:
- Dashboard Home – A financial summary with key metrics.
- Transactions – A table listing all expenses and incomes.
- Budgeting & Savings – A tool for setting budget goals.
- Analytics & Reports – Graphs and charts to track spending trends.
2. Design the Layout with HTML
Use HTML to structure your dashboard. Utilize elements like:
- <header> for the navigation bar.
- <aside> for the sidebar with menu links.
- <section> for different financial categories.
- <footer> for additional details or links.
3. Style the Dashboard with CSS
CSS enhances the visual appeal of your finance dashboard by:
- Using a clean and modern color scheme (blue and green are great for financial themes).
- Making the layout responsive with CSS Flexbox or Grid.
- Styling tables and charts for better readability.
- Adding hover effects for interactive elements.
4. Ensure Responsiveness for All Devices
A good finance dashboard should be mobile-friendly. Use CSS media queries to adjust layouts for different screen sizes, ensuring a seamless experience across desktops, tablets, and smartphones.
5. Optimize for Performance and SEO
To improve the efficiency and search ranking of your finance dashboard:
- Use SEO-friendly headings and meta descriptions.
- Optimize images to reduce load time.
- Minify CSS files for better performance.
- Use structured data to enhance search engine visibility.
Best Practices for an Effective Finance Dashboard
- Keep it simple and user-friendly for better navigation.
- Use high-contrast colors for easy readability.
- Update data dynamically for real-time insights.
- Include security features to protect financial data.
Source Code
- Step 1: Setting Up the HTML Structure
- Step 2: Adding Styling with CSS
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8" /><meta name="viewport" content="width=device-width, initial-scale=1.0" /><title>Premium Finance Dashboard</title><link rel="stylesheet" href="style.css" /></head><body><div class="container"><!-- Sidebar --><aside class="sidebar"><div class="sidebar-header"><div class="sidebar-logo"></div><h2>FinancePro</h2></div><div class="sidebar-section">Overview</div><ul class="nav-menu"><li class="nav-item"><a href="#" class="nav-link active"><span class="icon dashboard-icon"></span><span class="label">Dashboard</span></a></li><li class="nav-item"><a href="#" class="nav-link"><span class="icon wallet-icon"></span><span class="label">My Accounts</span></a></li><li class="nav-item"><a href="#" class="nav-link"><span class="icon transactions-icon"></span><span class="label">Transactions</span></a></li></ul><div class="sidebar-divider"></div><div class="sidebar-section">Management</div><ul class="nav-menu"><li class="nav-item"><a href="#" class="nav-link"><span class="icon investments-icon"></span><span class="label">Investments</span></a></li><li class="nav-item"><a href="#" class="nav-link"><span class="icon analytics-icon"></span><span class="label">Analytics</span></a></li><li class="nav-item"><a href="#" class="nav-link"><span class="icon savings-icon"></span><span class="label">Budgeting</span></a></li></ul><div class="sidebar-divider"></div><div class="sidebar-section">Settings</div><ul class="nav-menu"><li class="nav-item"><a href="#" class="nav-link"><span class="icon settings-icon"></span><span class="label">Settings</span></a></li></ul><div class="user-profile"><div class="user-avatar">JD</div><div class="user-info"><div class="user-name">John Doe</div><div class="user-role">Premium Account</div></div><div class="user-menu">⋮</div></div></aside><div class="overlay"></div><button class="menu-toggle">☰</button><!-- Main Content --><main class="main-content"><header class="page-header"><div><h1 class="page-title">Financial Dashboard</h1><p class="page-subtitle">Keep track of your financial health and transactions</p></div><div class="header-actions"><div class="search-box"><span class="search-icon"></span><inputtype="text"class="search-input"placeholder="Search for transactions..."/></div><div class="notification-wrapper"><button class="btn btn-secondary"><span class="icon notification-icon"></span><span class="badge">3</span></button></div><button class="btn btn-primary"><span class="icon add-icon"></span>New Transaction</button></div></header><div class="dashboard-grid"><div class="card"><div class="gradient-overlay gradient-primary"></div><div class="card-header"><h3 class="card-title">Total Balance</h3><div class="card-icon icon-primary"><span class="icon money-icon"></span></div></div><div class="card-value">$24,562.00</div><div class="card-change"><span class="positive">↑ 5.2%</span> from last month</div></div><div class="card"><div class="gradient-overlay gradient-success"></div><div class="card-header"><h3 class="card-title">Income</h3><div class="card-icon icon-success"><span class="icon income-icon"></span></div></div><div class="card-value">$8,942.00</div><div class="card-change"><span class="positive">↑ 2.5%</span> from last month</div></div><div class="card"><div class="gradient-overlay gradient-danger"></div><div class="card-header"><h3 class="card-title">Expenses</h3><div class="card-icon icon-danger"><span class="icon expense-icon"></span></div></div><div class="card-value">$5,325.00</div><div class="card-change"><span class="negative">↑ 1.8%</span> from last month</div></div><div class="card chart-container"><div class="chart-header"><div class="chart-tabs"><div class="chart-tab active">Monthly</div><div class="chart-tab">Quarterly</div><div class="chart-tab">Yearly</div></div></div><svgviewBox="0 0 800 300"xmlns="http://www.w3.org/2000/svg"style="width: 100%; height: 300px"><!-- Grid Lines --><linex1="50"y1="250"x2="750"y2="250"stroke="#f1f5f9"stroke-width="1"/><linex1="50"y1="200"x2="750"y2="200"stroke="#f1f5f9"stroke-width="1"/><linex1="50"y1="150"x2="750"y2="150"stroke="#f1f5f9"stroke-width="1"/><linex1="50"y1="100"x2="750"y2="100"stroke="#f1f5f9"stroke-width="1"/><linex1="50"y1="50"x2="750"y2="50"stroke="#f1f5f9"stroke-width="1"/><defs><linearGradientid="incomeGradient"x1="0%"y1="0%"x2="0%"y2="100%"><stopoffset="0%"style="stop-color: #10b981; stop-opacity: 0.3"/><stopoffset="100%"style="stop-color: #10b981; stop-opacity: 0"/></linearGradient></defs><pathd="M 50 160L 152 140L 254 150L 356 120L 458 100L 560 90L 662 70L 750 60L 750 250L 50 250 Z"fill="url(#incomeGradient)"/><pathd="M 50 160L 152 140L 254 150L 356 120L 458 100L 560 90L 662 70L 750 60"fill="none"stroke="#10b981"stroke-width="3"stroke-linecap="round"stroke-linejoin="round"/><circle cx="50" cy="160" r="5" fill="#10b981" /><circle cx="152" cy="140" r="5" fill="#10b981" /><circle cx="254" cy="150" r="5" fill="#10b981" /><circle cx="356" cy="120" r="5" fill="#10b981" /><circle cx="458" cy="100" r="5" fill="#10b981" /><circle cx="560" cy="90" r="5" fill="#10b981" /><circle cx="662" cy="70" r="5" fill="#10b981" /><circle cx="750" cy="60" r="5" fill="#10b981" /><defs><linearGradientid="expenseGradient"x1="0%"y1="0%"x2="0%"y2="100%"><stopoffset="0%"style="stop-color: #ef4444; stop-opacity: 0.3"/><stopoffset="100%"style="stop-color: #ef4444; stop-opacity: 0"/></linearGradient></defs><pathd="M 50 200L 152 190L 254 210L 356 180L 458 170L 560 180L 662 150L 750 160L 750 250L 50 250 Z"fill="url(#expenseGradient)"/><pathd="M 50 200L 152 190L 254 210L 356 180L 458 170L 560 180L 662 150L 750 160"fill="none"stroke="#ef4444"stroke-width="3"stroke-linecap="round"stroke-linejoin="round"/><circle cx="50" cy="200" r="5" fill="#ef4444" /><circle cx="152" cy="190" r="5" fill="#ef4444" /><circle cx="254" cy="210" r="5" fill="#ef4444" /><circle cx="356" cy="180" r="5" fill="#ef4444" /><circle cx="458" cy="170" r="5" fill="#ef4444" /><circle cx="560" cy="180" r="5" fill="#ef4444" /><circle cx="662" cy="150" r="5" fill="#ef4444" /><circle cx="750" cy="160" r="5" fill="#ef4444" /><textx="50"y="275"text-anchor="middle"font-size="12"fill="#6b7280">Jan</text><textx="152"y="275"text-anchor="middle"font-size="12"fill="#6b7280">Feb</text><textx="254"y="275"text-anchor="middle"font-size="12"fill="#6b7280">Mar</text><textx="356"y="275"text-anchor="middle"font-size="12"fill="#6b7280">Apr</text><textx="458"y="275"text-anchor="middle"font-size="12"fill="#6b7280">May</text><textx="560"y="275"text-anchor="middle"font-size="12"fill="#6b7280">Jun</text><textx="662"y="275"text-anchor="middle"font-size="12"fill="#6b7280">Jul</text><textx="750"y="275"text-anchor="middle"font-size="12"fill="#6b7280">Aug</text><textx="35"y="250"text-anchor="end"font-size="12"fill="#6b7280">$0k</text><textx="35"y="200"text-anchor="end"font-size="12"fill="#6b7280">$2k</text><textx="35"y="150"text-anchor="end"font-size="12"fill="#6b7280">$4k</text><textx="35"y="100"text-anchor="end"font-size="12"fill="#6b7280">$6k</text><textx="35"y="50"text-anchor="end"font-size="12"fill="#6b7280">$8k</text><!-- Legend --><circle cx="650" cy="30" r="5" fill="#10b981" /><textx="660"y="35"font-size="14"fill="#111827"font-weight="500">Income</text><circle cx="730" cy="30" r="5" fill="#ef4444" /><textx="740"y="35"font-size="14"fill="#111827"font-weight="500">Expenses</text></svg></div><div class="card transactions"><div class="card-header"><h3 class="card-title">Recent Transactions</h3><a href="#" class="view-all">View All <span class="icon arrow-right"></span></a></div><div class="table-container"><table class="table"><thead><tr><th>TRANSACTION</th><th>CATEGORY</th><th>DATE</th><th>AMOUNT</th><th>STATUS</th></tr></thead><tbody><tr><td><div class="transaction-user"><div class="transaction-icon rent">🏠</div><div class="transaction-details"><div class="transaction-name">Monthly Rent</div><div class="transaction-date">11:15 AM</div></div></div></td><td>Housing</td><td>Mar 15, 2025</td><td><span class="amount amount-negative">-$1,500.00</span></td><td><span class="status status-completed">Completed</span></td></tr><tr><td><div class="transaction-user"><div class="transaction-icon salary">💼</div><div class="transaction-details"><div class="transaction-name">Salary Deposit</div><div class="transaction-date">09:32 AM</div></div></div></td><td>Income</td><td>Mar 10, 2025</td><td><span class="amount amount-positive">+$4,300.00</span></td><td><span class="status status-completed">Completed</span></td></tr><tr><td><div class="transaction-user"><div class="transaction-icon subscription">📺</div><div class="transaction-details"><div class="transaction-name">Netflix Subscription</div><div class="transaction-date">06:11 PM</div></div></div></td><td>Entertainment</td><td>Mar 08, 2025</td><td><span class="amount amount-negative">-$14.99</span></td><td><span class="status status-completed">Completed</span></td></tr><tr><td><div class="transaction-user"><div class="transaction-icon grocery">🛒</div><div class="transaction-details"><div class="transaction-name">Grocery Shopping</div><div class="transaction-date">03:45 PM</div></div></div></td><td>Food</td><td>Mar 05, 2025</td><td><span class="amount amount-negative">-$120.50</span></td><td><span class="status status-completed">Completed</span></td></tr><tr><td><div class="transaction-user"><div class="transaction-icon investment">📊</div><div class="transaction-details"><div class="transaction-name">Stock Investment</div><div class="transaction-date">11:36 AM</div></div></div></td><td>Investment</td><td>Mar 01, 2025</td><td><span class="amount amount-negative">-$500.00</span></td><td><span class="status status-pending">Pending</span></td></tr></tbody></table></div></div></div><div class="analytics-section"><div class="section-header"><h2 class="section-title">Spending Analytics</h2><a href="#" class="view-all">View Detailed Report <span class="icon arrow-right"></span></a></div><div class="dashboard-grid"><div class="card"><div class="card-header"><h3 class="card-title">Spending by Category</h3></div><svgviewBox="0 0 400 200"xmlns="http://www.w3.org/2000/svg"style="width: 100%; height: 200px"><g transform="translate(100, 100)"><pathd="M 0 0 L 0 -80 A 80 80 0 0 1 69.28 -40 Z"fill="#6366f1"/><pathd="M 0 0 L 69.28 -40 A 80 80 0 0 1 80 0 Z"fill="#10b981"/><pathd="M 0 0 L 80 0 A 80 80 0 0 1 40 69.28 Z"fill="#f59e0b"/><pathd="M 0 0 L 40 69.28 A 80 80 0 0 1 -27.71 76.05 Z"fill="#ef4444"/><pathd="M 0 0 L -27.71 76.05 A 80 80 0 0 1 -80 0 Z"fill="#8b5cf6"/><circle cx="0" cy="0" r="50" fill="white" /></g><!-- Legend --><g transform="translate(220, 40)"><rect x="0" y="0" width="12" height="12" fill="#6366f1" /><text x="20" y="10" font-size="12" fill="#111827">Housing (35%)</text><rect x="0" y="25" width="12" height="12" fill="#10b981" /><text x="20" y="35" font-size="12" fill="#111827">Food (25%)</text><rect x="0" y="50" width="12" height="12" fill="#f59e0b" /><text x="20" y="60" font-size="12" fill="#111827">Transportation (15%)</text><rect x="0" y="75" width="12" height="12" fill="#ef4444" /><text x="20" y="85" font-size="12" fill="#111827">Entertainment (10%)</text><rect x="0" y="100" width="12" height="12" fill="#8b5cf6" /><text x="20" y="110" font-size="12" fill="#111827">Others (15%)</text></g></svg></div><div class="card"><div class="card-header"><h3 class="card-title">Savings Goal</h3></div><div style="padding: 20px 0"><div style="text-align: center; margin-bottom: 15px"><divstyle="font-size: 36px;font-weight: 700;color: var(--text-primary);">$8,450</div><div style="color: var(--text-muted); margin-top: 5px">saved of $10,000 goal</div></div><!-- Progress Bar --><divstyle="width: 100%;height: 10px;background-color: #f1f5f9;border-radius: 10px;margin: 30px 0;"><divstyle="width: 84.5%;height: 10px;background: linear-gradient(to right, #6366f1, #8b5cf6);border-radius: 10px;"></div></div><divstyle="display: flex;justify-content: space-between;margin-top: 10px;"><div style="font-weight: 600; color: var(--success)">84.5% Complete</div><div style="color: var(--text-muted)">$1,550 to go</div></div></div></div><div class="card"><div class="card-header"><h3 class="card-title">Upcoming Bills</h3></div><div style="margin-top: 15px"><divstyle="display: flex;align-items: center;justify-content: space-between;padding: 12px 0;border-bottom: 1px solid #f1f5f9;"><div style="display: flex; align-items: center; gap: 12px"><divstyle="width: 40px;height: 40px;border-radius: 12px;background-color: rgba(239, 68, 68, 0.1);color: #ef4444;display: flex;align-items: center;justify-content: center;font-size: 18px;">💳</div><div><div style="font-weight: 600; color: var(--text-primary)">Credit Card</div><div style="font-size: 12px; color: var(--text-muted)">Due in 3 days</div></div></div><div style="font-weight: 600; color: var(--text-primary)">$1,250.00</div></div><divstyle="display: flex;align-items: center;justify-content: space-between;padding: 12px 0;border-bottom: 1px solid #f1f5f9;"><div style="display: flex; align-items: center; gap: 12px"><divstyle="width: 40px;height: 40px;border-radius: 12px;background-color: rgba(14, 165, 233, 0.1);color: #0ea5e9;display: flex;align-items: center;justify-content: center;font-size: 18px;">💧</div><div><div style="font-weight: 600; color: var(--text-primary)">Water & Electricity</div><div style="font-size: 12px; color: var(--text-muted)">Due in 7 days</div></div></div><div style="font-weight: 600; color: var(--text-primary)">$145.50</div></div><divstyle="display: flex;align-items: center;justify-content: space-between;padding: 12px 0;"><div style="display: flex; align-items: center; gap: 12px"><divstyle="width: 40px;height: 40px;border-radius: 12px;background-color: rgba(245, 158, 11, 0.1);color: #f59e0b;display: flex;align-items: center;justify-content: center;font-size: 18px;">📱</div><div><div style="font-weight: 600; color: var(--text-primary)">Internet & Phone</div><div style="font-size: 12px; color: var(--text-muted)">Due in 12 days</div></div></div><div style="font-weight: 600; color: var(--text-primary)">$89.99</div></div></div></div></div></div></main></div><script>document.addEventListener("DOMContentLoaded", function () {const menuToggle = document.querySelector(".menu-toggle");const sidebar = document.querySelector(".sidebar");const overlay = document.querySelector(".overlay");if (menuToggle && sidebar && overlay) {menuToggle.addEventListener("click", function () {sidebar.classList.toggle("active");overlay.classList.toggle("active");});overlay.addEventListener("click", function () {sidebar.classList.remove("active");overlay.classList.remove("active");});}});</script></body></html>
:root {--primary: #6366f1;--primary-dark: #4f46e5;--secondary: #0ea5e9;--success: #10b981;--danger: #ef4444;--warning: #f59e0b;--dark: #111827;--dark-accent: #1f2937;--light: #f9fafb;--gray: #9ca3af;--text-primary: #111827;--text-secondary: #4b5563;--text-muted: #6b7280;--border-radius: 12px;--box-shadow: 0 10px 25px -5px rgba(0, 0, 0, 0.05);--transition: all 0.3s cubic-bezier(0.4, 0, 0.2, 1);--font-family: "Inter", -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto,Oxygen, Ubuntu, Cantarell, "Open Sans", "Helvetica Neue", sans-serif;}* {margin: 0;padding: 0;box-sizing: border-box;font-family: var(--font-family);}body {background-color: #f5f7fa;color: var(--text-primary);line-height: 1.6;font-size: 14px;}.container {display: grid;grid-template-columns: 280px 1fr;min-height: 100vh;}/* Glassmorphism effects */.glass {background: rgba(255, 255, 255, 0.6);backdrop-filter: blur(15px);-webkit-backdrop-filter: blur(15px);border: 1px solid rgba(255, 255, 255, 0.18);}/* Sidebar Styles */.sidebar {background: linear-gradient(135deg, var(--dark) 0%, var(--dark-accent) 100%);color: var(--light);padding: 30px 24px;position: fixed;top: 0;bottom: 0;left: 0;/* height: 100vh; */width: 280px;transition: var(--transition);z-index: 100;overflow-y: auto;overflow-x: hidden;}.sidebar-header {display: flex;align-items: center;margin-bottom: 40px;}.sidebar-header h2 {font-size: 24px;font-weight: 700;margin-left: 12px;background: linear-gradient(90deg, #6366f1, #8b5cf6);-webkit-background-clip: text;-webkit-text-fill-color: transparent;letter-spacing: -0.5px;}.sidebar-logo {display: flex;align-items: center;justify-content: center;width: 40px;height: 40px;background: linear-gradient(135deg,var(--primary) 0%,var(--primary-dark) 100%);border-radius: 12px;box-shadow: 0 5px 15px rgba(99, 102, 241, 0.4);}.sidebar-divider {height: 1px;background: rgba(255, 255, 255, 0.1);margin: 20px 0;}.sidebar-section {margin-bottom: 8px;font-size: 12px;text-transform: uppercase;letter-spacing: 1px;color: var(--gray);padding-left: 14px;}.nav-menu {list-style: none;margin-top: 10px;}.nav-item {margin-bottom: 5px;}.nav-link {display: flex;align-items: center;padding: 12px 16px;border-radius: var(--border-radius);color: rgba(255, 255, 255, 0.7);text-decoration: none;transition: var(--transition);font-weight: 500;}.nav-link i {font-size: 20px;margin-right: 12px;}.nav-link span.label {flex: 1;}.nav-link.active {background: linear-gradient(90deg,rgba(99, 102, 241, 0.2),rgba(99, 102, 241, 0));color: white;}.nav-link.active::before {content: "";position: absolute;left: 0;width: 4px;height: 24px;background: var(--primary);border-radius: 0 4px 4px 0;}.nav-link:hover:not(.active) {background-color: rgba(255, 255, 255, 0.05);color: white;}.user-profile {position: absolute;bottom: 20px;left: 24px;right: 24px;display: flex;align-items: center;padding: 16px;border-radius: var(--border-radius);background-color: rgba(255, 255, 255, 0.05);border: 1px solid rgba(255, 255, 255, 0.08);transition: var(--transition);cursor: pointer;}.user-profile:hover {background-color: rgba(255, 255, 255, 0.1);}.user-avatar {width: 42px;height: 42px;border-radius: 50%;background: linear-gradient(135deg, #60a5fa, #3b82f6);margin-right: 12px;box-shadow: 0 5px 15px rgba(59, 130, 246, 0.4);display: flex;align-items: center;justify-content: center;font-size: 18px;font-weight: 600;color: white;}.user-info {flex: 1;}.user-name {font-weight: 600;font-size: 14px;color: white;}.user-role {color: var(--gray);font-size: 12px;margin-top: 2px;}.user-menu {color: var(--gray);font-size: 18px;}/* Main Content */.main-content {grid-column: 2;padding: 30px 40px;}.page-header {display: flex;justify-content: space-between;align-items: center;margin-bottom: 30px;position: relative;}.page-title {font-size: 26px;font-weight: 700;color: var(--text-primary);letter-spacing: -0.5px;}.page-subtitle {color: var(--text-muted);font-size: 14px;margin-top: 4px;}.header-actions {display: flex;gap: 16px;align-items: center;}.btn {padding: 12px 24px;border-radius: var(--border-radius);border: none;cursor: pointer;transition: var(--transition);font-weight: 500;font-size: 14px;display: flex;align-items: center;justify-content: center;gap: 8px;}.btn-primary {background: linear-gradient(to right, var(--primary), var(--primary-dark));color: white;box-shadow: 0 6px 12px -2px rgba(99, 102, 241, 0.3);}.btn-primary:hover {box-shadow: 0 8px 16px -2px rgba(99, 102, 241, 0.4);transform: translateY(-2px);}.btn-secondary {background-color: white;color: var(--text-primary);border: 1px solid #e5e7eb;}.btn-secondary:hover {background-color: #f9fafb;box-shadow: var(--box-shadow);}.search-box {position: relative;}.search-input {padding: 12px 20px 12px 48px;border-radius: var(--border-radius);border: 1px solid #e5e7eb;width: 280px;transition: var(--transition);font-size: 14px;background-color: white;}.search-input:focus {outline: none;box-shadow: 0 0 0 2px rgba(99, 102, 241, 0.2);border-color: var(--primary);}.search-icon {position: absolute;left: 18px;top: 50%;transform: translateY(-50%);color: var(--text-muted);font-size: 18px;}/* Dashboard Grid */.dashboard-grid {display: grid;grid-template-columns: repeat(3, 1fr);grid-template-rows: auto auto;gap: 24px;margin-bottom: 24px;}.card {background-color: white;border-radius: var(--border-radius);box-shadow: var(--box-shadow);padding: 20px;transition: var(--transition);position: relative;overflow: hidden;border: 1px solid rgba(0, 0, 0, 0.05);}.card:hover {transform: translateY(-5px);box-shadow: 0 15px 30px rgba(0, 0, 0, 0.08);}.gradient-overlay {position: absolute;top: 0;right: 0;width: 100px;height: 100%;opacity: 0.1;transition: var(--transition);}.gradient-primary {background: linear-gradient(135deg, transparent, var(--primary));}.gradient-success {background: linear-gradient(135deg, transparent, var(--success));}.gradient-danger {background: linear-gradient(135deg, transparent, var(--danger));}.card:hover .gradient-overlay {opacity: 0.15;}.card-header {display: flex;justify-content: space-between;align-items: center;margin-bottom: 20px;}.card-title {font-size: 15px;font-weight: 600;color: var(--text-secondary);}.card-icon {width: 48px;height: 48px;border-radius: 50%;display: flex;align-items: center;justify-content: center;color: white;font-size: 20px;box-shadow: 0 10px 20px rgba(0, 0, 0, 0.1);}.icon-primary {background: linear-gradient(135deg, var(--primary), var(--primary-dark));}.icon-success {background: linear-gradient(135deg, var(--success), #059669);}.icon-danger {background: linear-gradient(135deg, var(--danger), #dc2626);}.icon-warning {background: linear-gradient(135deg, var(--warning), #d97706);}.card-value {font-size: 36px;font-weight: 700;margin-bottom: 8px;letter-spacing: -0.5px;color: var(--text-primary);}.card-change {font-size: 14px;display: flex;align-items: center;color: var(--text-muted);gap: 5px;}.positive {color: var(--success);font-weight: 600;}.negative {color: var(--danger);font-weight: 600;}/* Charts */.chart-container {grid-column: 1 / -1;height: 400px;position: relative;}.placeholder-chart {width: 100%;height: 100%;display: flex;align-items: center;justify-content: center;border-radius: var(--border-radius);background: linear-gradient(135deg, #f8fafc 0%, #f1f5f9 100%);font-size: 16px;color: var(--text-muted);border: 1px dashed #cbd5e1;}.chart-tabs {display: flex;margin-bottom: 20px;gap: 10px;}.chart-tab {padding: 8px 16px;border-radius: var(--border-radius);background-color: #f1f5f9;cursor: pointer;transition: var(--transition);font-size: 13px;font-weight: 500;}.chart-tab.active {background: linear-gradient(to right, var(--primary), var(--primary-dark));color: white;box-shadow: 0 6px 12px -2px rgba(99, 102, 241, 0.3);}/* Table Styles */.transactions {grid-column: 1 / -1;}.table-container {overflow-x: auto;border-radius: var(--border-radius);}.table {width: 100%;border-collapse: separate;border-spacing: 0;}.table th {padding: 16px 20px;text-align: left;font-weight: 600;color: var(--text-secondary);background-color: #f8fafc;font-size: 13px;text-transform: uppercase;letter-spacing: 0.5px;}.table th:first-child {border-top-left-radius: var(--border-radius);}.table th:last-child {border-top-right-radius: var(--border-radius);}.table td {padding: 16px 20px;font-size: 14px;border-bottom: 1px solid #f1f5f9;}.table tr:last-child td {border-bottom: none;}.table tr:last-child td:first-child {border-bottom-left-radius: var(--border-radius);}.table tr:last-child td:last-child {border-bottom-right-radius: var(--border-radius);}.table tr:hover td {background-color: #f8fafc;}.transaction-user {display: flex;align-items: center;gap: 12px;}.transaction-icon {width: 40px;height: 40px;border-radius: 12px;background-color: #f1f5f9;display: flex;align-items: center;justify-content: center;font-size: 18px;}.transaction-icon.rent {color: #8b5cf6;background-color: rgba(139, 92, 246, 0.1);}.transaction-icon.salary {color: #10b981;background-color: rgba(16, 185, 129, 0.1);}.transaction-icon.subscription {color: #f43f5e;background-color: rgba(244, 63, 94, 0.1);}.transaction-icon.grocery {color: #f59e0b;background-color: rgba(245, 158, 11, 0.1);}.transaction-icon.investment {color: #3b82f6;background-color: rgba(59, 130, 246, 0.1);}.transaction-details {flex: 1;}.transaction-name {font-weight: 600;margin-bottom: 3px;color: var(--text-primary);}.transaction-date {font-size: 12px;color: var(--text-muted);}.amount {font-weight: 600;font-size: 15px;}.amount-negative {color: var(--danger);}.amount-positive {color: var(--success);}.status {padding: 6px 12px;border-radius: 20px;font-size: 12px;font-weight: 500;display: inline-block;}.status-completed {background-color: rgba(16, 185, 129, 0.1);color: var(--success);}.status-pending {background-color: rgba(245, 158, 11, 0.1);color: var(--warning);}.status-failed {background-color: rgba(239, 68, 68, 0.1);color: var(--danger);}/* Analytics cards */.analytics-section {margin-bottom: 30px;}.section-header {display: flex;justify-content: space-between;align-items: center;margin-bottom: 20px;}.section-title {font-size: 18px;font-weight: 700;color: var(--text-primary);}.view-all {color: var(--primary);font-weight: 500;text-decoration: none;font-size: 14px;display: flex;align-items: center;gap: 5px;}.view-all:hover {text-decoration: underline;}/* Custom scrollbar */::-webkit-scrollbar {width: 8px;height: 8px;}::-webkit-scrollbar-track {background: #f1f5f9;}::-webkit-scrollbar-thumb {background: #cbd5e1;border-radius: 10px;}::-webkit-scrollbar-thumb:hover {background: #94a3b8;}/* Responsive Design */@media (max-width: 1280px) {.dashboard-grid {grid-template-columns: repeat(2, 1fr);}}@media (max-width: 1024px) {.container {grid-template-columns: 1fr;}.sidebar {width: 0;padding: 0;overflow: hidden;opacity: 0;}.sidebar.active {width: 280px;padding: 30px 24px;opacity: 1;}.main-content {margin-left: 0;padding: 20px;}.search-input {width: 100%;max-width: 280px;}}@media (max-width: 768px) {.dashboard-grid {grid-template-columns: 1fr;}.header-actions {flex-direction: column;align-items: flex-start;gap: 10px;}.page-header {flex-direction: column;align-items: flex-start;gap: 15px;}.search-input {width: 100%;}.card {padding: 20px;}}/* Menu toggle for mobile */.menu-toggle {background: none;border: none;color: var(--text-primary);font-size: 24px;cursor: pointer;display: none;z-index: 200;}@media (max-width: 1024px) {.menu-toggle {display: block;position: fixed;top: 20px;right: 20px;background: white;border-radius: 50%;width: 40px;height: 40px;display: flex;align-items: center;justify-content: center;box-shadow: var(--box-shadow);}.overlay {position: fixed;top: 0;left: 0;right: 0;bottom: 0;background: rgba(0, 0, 0, 0.5);z-index: 90;opacity: 0;pointer-events: none;transition: var(--transition);}.overlay.active {opacity: 1;pointer-events: auto;}}/* Icons */.icon {display: flex;align-items: center;justify-content: center;}.dashboard-icon:before {content: "📊";}.wallet-icon:before {content: "💰";}.transactions-icon:before {content: "🔄";}.investments-icon:before {content: "📈";}.analytics-icon:before {content: "📉";}.settings-icon:before {content: "⚙️";}.search-icon:before {content: "🔍";}.money-icon:before {content: "💵";}.expense-icon:before {content: "💸";}.savings-icon:before {content: "🏦";}.income-icon:before {content: "📥";}.notification-icon:before {content: "🔔";}.arrow-right:before {content: "→";}.add-icon:before {content: "+";}.badge {position: absolute;top: -5px;right: -5px;width: 18px;height: 18px;border-radius: 50%;background-color: var(--danger);color: white;font-size: 10px;font-weight: 600;display: flex;align-items: center;justify-content: center;border: 2px solid white;}.notification-wrapper {position: relative;}
Conclusion
A well-designed finance dashboard is an invaluable tool for managing and analyzing financial data efficiently. By leveraging HTML and CSS, you can create an interactive and visually appealing dashboard that helps users stay on top of their finances. Start building your finance dashboard today and take control of your financial future!
Web Developer and Content Creator