Make a Crypto Dashboard with HTML and CSS
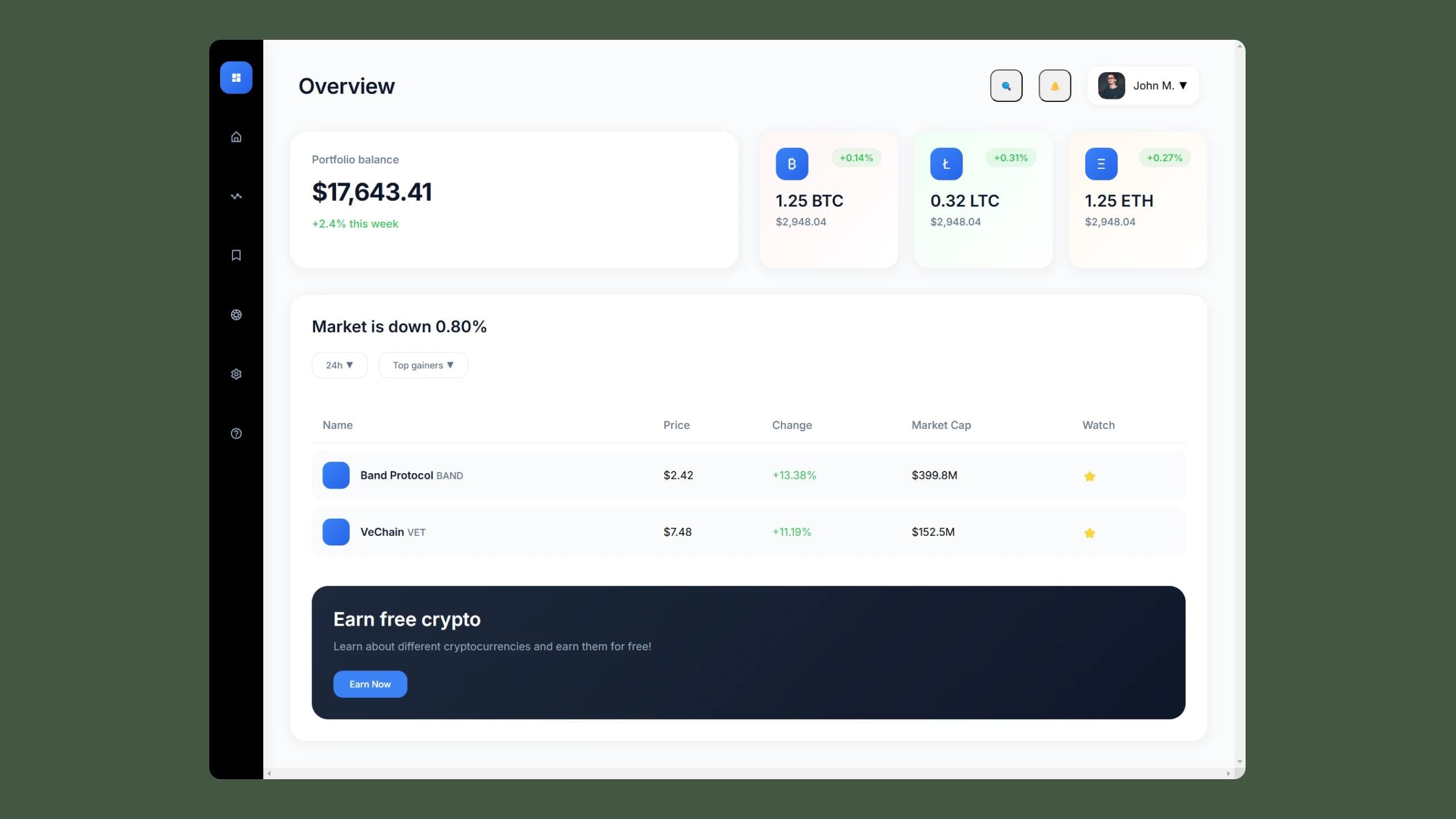
A crypto dashboard built with HTML and CSS helps users monitor cryptocurrency prices, track portfolio balances, and access market insights in real time. With an intuitive layout and responsive design, you can create a user-friendly and visually appealing interface for tracking crypto assets.
Why Use HTML and CSS for a Crypto Dashboard?
HTML and CSS provide the foundational elements for structuring and styling a web-based dashboard. They enable developers to craft interactive and adaptive designs without relying on complex frameworks. Check out finance dashboard with HTML and CSS
Key Features of a Crypto Dashboard
- Portfolio Overview: Display real-time balance and asset performance.
- Market Data: Show price changes, trends, and market capitalization.
- Navigation Sidebar: Ensure seamless access to different sections.
- Responsive Design: Adapt to various screen sizes with CSS media queries.
Building a crypto dashboard with HTML and CSS is a practical approach to designing an interactive and responsive interface. By structuring the layout with HTML and enhancing aesthetics with CSS, you can create an effective tool for tracking cryptocurrency data.
Steps to Build a Crypto Dashboard with HTML and CSS
Setting Up the HTML Structure
Start by structuring the dashboard with semantic HTML elements like <div>, <nav>, and <section>.
Styling with CSS
Enhance the dashboard's visual appeal using CSS.
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8" /><meta name="viewport" content="width=device-width, initial-scale=1.0" /><title>Crypto Dashboard</title><linkhref="https://unpkg.com/boxicons@2.1.4/css/boxicons.min.css"rel="stylesheet"/><link rel="stylesheet" href="style.css" /></head><body><div class="dashboard"><aside class="sidebar"><div class="logo"><i class="bx bxs-dashboard"></i></div><div class="nav-item"><i class="bx bx-home-alt"></i></div><div class="nav-item"><i class="bx bx-stats"></i></div><div class="nav-item"><i class="bx bx-bookmark"></i></div><div class="nav-item"><i class="bx bx-color"></i></div><div class="nav-item"><i class="bx bx-cog"></i></div><div class="nav-item"><i class="bx bx-help-circle"></i></div></aside><main class="main-content"><header class="header"><div class="header-left"><h1>Overview</h1></div><div class="header-right"><button class="nav-item">🔍</button><button class="nav-item">🔔</button><div class="user-profile"><img src="./images/user.jpg" alt="Profile" class="avatar" /><span>John M. ▼</span></div></div></header><section class="portfolio-section"><div class="balance-card"><div class="balance-header"><div><p style="color: #64748b; font-weight: 500">Portfolio balance</p><h2 class="balance-amount">$17,643.41</h2><p style="color: #34c759; font-weight: 500">+2.4% this week</p></div></div></div><div class="assets-grid"><div class="asset-card btc"><div class="crypto-icon">₿</div><div class="asset-amount">1.25 BTC</div><div class="asset-value">$2,948.04</div><div class="change-indicator positive">+0.14%</div></div><div class="asset-card ltc"><div class="crypto-icon">Ł</div><div class="asset-amount">0.32 LTC</div><div class="asset-value">$2,948.04</div><div class="change-indicator positive">+0.31%</div></div><div class="asset-card eth"><div class="crypto-icon">Ξ</div><div class="asset-amount">1.25 ETH</div><div class="asset-value">$2,948.04</div><div class="change-indicator positive">+0.27%</div></div></div></section><section class="market-section"><h2>Market is down 0.80%</h2><div class="market-filters"><button class="filter-button">24h ▼</button><button class="filter-button">Top gainers ▼</button></div><table class="market-table"><thead><tr><th>Name</th><th>Price</th><th>Change</th><th>Market Cap</th><th>Watch</th></tr></thead><tbody><tr><td><div class="coin-cell"><div class="coin-icon"></div><div class="coin-info"><span class="coin-name">Band Protocol</span><span class="coin-symbol">BAND</span></div></div></td><td>$2.42</td><td class="positive">+13.38%</td><td>$399.8M</td><td>⭐</td></tr><tr><td><div class="coin-cell"><div class="coin-icon"></div><div class="coin-info"><span class="coin-name">VeChain</span><span class="coin-symbol">VET</span></div></div></td><td>$7.48</td><td class="positive">+11.19%</td><td>$152.5M</td><td>⭐</td></tr></tbody></table><div class="promo-card"><h3>Earn free crypto</h3><p>Learn about different cryptocurrencies and earn them for free!</p><button class="promo-button">Earn Now</button></div></section></main></div></body></html>
@import url("https://fonts.googleapis.com/css2?family=Inter:wght@300;400;500;600;700&display=swap");* {margin: 0;padding: 0;box-sizing: border-box;font-family: "Inter", sans-serif;}body {background: #f8fafc;}.dashboard {display: flex;min-height: 100vh;background: #f8fafc;}.sidebar {width: 80px;height: 100%;position: fixed;top: 0;bottom: 0;background: #000000;padding: 32px 16px;display: flex;flex-direction: column;align-items: center;gap: 40px;box-shadow: 4px 0 20px rgba(0, 0, 0, 0.05);}.logo {width: 48px;height: 48px;display: flex;align-items: center;justify-content: center;color: white;background: linear-gradient(135deg, #3b82f6, #2563eb);border-radius: 14px;}.nav-item {width: 48px;height: 48px;display: flex;align-items: center;justify-content: center;color: #94a3b8;border-radius: 12px;cursor: pointer;transition: all 0.2s ease;}.nav-item i {font-size: 1.2rem;}.nav-item:hover {background: rgba(255, 255, 255, 0.1);color: #ffffff;transform: translateY(-2px);}.main-content {margin-left: 80px;flex: 1;padding: 40px;max-width: 1600px;overflow: scroll;}.header {display: flex;justify-content: space-between;align-items: center;margin-bottom: 40px;padding: 0 12px;}.header-left h1 {font-size: 32px;font-weight: 600;color: #0f172a;letter-spacing: -0.5px;}.header-right {display: flex;align-items: center;gap: 24px;}.user-profile {display: flex;align-items: center;gap: 12px;padding: 8px 16px;background: white;border-radius: 16px;box-shadow: 0 2px 10px rgba(0, 0, 0, 0.05);}.avatar {width: 40px;height: 40px;border-radius: 12px;object-fit: cover;}.portfolio-section {display: grid;grid-template-columns: 1fr 1fr;gap: 32px;margin-bottom: 40px;}.balance-card {background: white;padding: 32px;border-radius: 24px;box-shadow: 0 4px 20px rgba(0, 0, 0, 0.05);}.balance-header {display: flex;justify-content: space-between;margin-bottom: 24px;}.balance-amount {font-size: 36px;font-weight: 700;color: #0f172a;letter-spacing: -1px;margin: 16px 0;}.assets-grid {display: grid;grid-template-columns: repeat(3, 1fr);gap: 24px;}.asset-card {background: white;padding: 24px;border-radius: 20px;position: relative;box-shadow: 0 4px 20px rgba(0, 0, 0, 0.05);transition: transform 0.2s ease;}.asset-card:hover {transform: translateY(-4px);}.asset-card.btc {background: linear-gradient(135deg, #fff5f5 0%, #ffffff 100%);}.asset-card.ltc {background: linear-gradient(135deg, #f0fff4 0%, #ffffff 100%);}.asset-card.eth {background: linear-gradient(135deg, #fff8f0 0%, #ffffff 100%);}.crypto-icon {width: 48px;height: 48px;border-radius: 14px;background: linear-gradient(135deg, #3b82f6, #2563eb);color: white;display: flex;align-items: center;justify-content: center;font-size: 20px;margin-bottom: 16px;}.asset-amount {font-size: 24px;font-weight: 600;color: #0f172a;margin-bottom: 8px;}.asset-value {color: #64748b;font-size: 15px;font-weight: 500;}.change-indicator {position: absolute;top: 24px;right: 24px;font-size: 14px;font-weight: 600;padding: 6px 12px;border-radius: 20px;background: rgba(52, 199, 89, 0.1);}.positive {color: #34c759;}.market-section {background: white;padding: 32px;border-radius: 24px;box-shadow: 0 4px 20px rgba(0, 0, 0, 0.05);}.market-section h2 {font-size: 24px;color: #0f172a;margin-bottom: 24px;font-weight: 600;}.market-filters {display: flex;gap: 16px;margin-bottom: 32px;}.filter-button {padding: 10px 20px;border-radius: 14px;border: 1px solid #e2e8f0;background: white;color: #64748b;font-weight: 500;cursor: pointer;transition: all 0.2s ease;}.filter-button:hover {background: #f8fafc;border-color: #cbd5e1;}.market-table {width: 100%;border-collapse: separate;border-spacing: 0 12px;}.market-table th {text-align: left;padding: 16px;color: #64748b;font-weight: 500;border-bottom: 1px solid #e2e8f0;}.market-table td {padding: 16px;background: #f8fafc;border-top: none;}.market-table tr td:first-child {border-top-left-radius: 12px;border-bottom-left-radius: 12px;}.market-table tr td:last-child {border-top-right-radius: 12px;border-bottom-right-radius: 12px;}.coin-cell {display: flex;align-items: center;gap: 16px;}.coin-icon {width: 40px;height: 40px;border-radius: 12px;background: linear-gradient(135deg, #3b82f6, #2563eb);}.coin-name {font-weight: 600;color: #0f172a;}.coin-symbol {color: #64748b;font-size: 14px;font-weight: 500;}.promo-card {background: linear-gradient(135deg, #1e293b, #0f172a);padding: 32px;border-radius: 24px;margin-top: 32px;}.promo-card h3 {font-size: 28px;color: white;margin-bottom: 12px;font-weight: 600;}.promo-card p {color: #94a3b8;margin-bottom: 24px;font-size: 16px;line-height: 1.5;}.promo-button {background: #3b82f6;color: white;padding: 12px 24px;border-radius: 14px;border: none;font-weight: 500;cursor: pointer;transition: all 0.2s ease;}.promo-button:hover {background: #2563eb;transform: translateY(-2px);}
Web Developer and Content Creator